Image Carousel Solution
Recap
Hopefully you had success re-creating the JavaScript image carousel challenge!
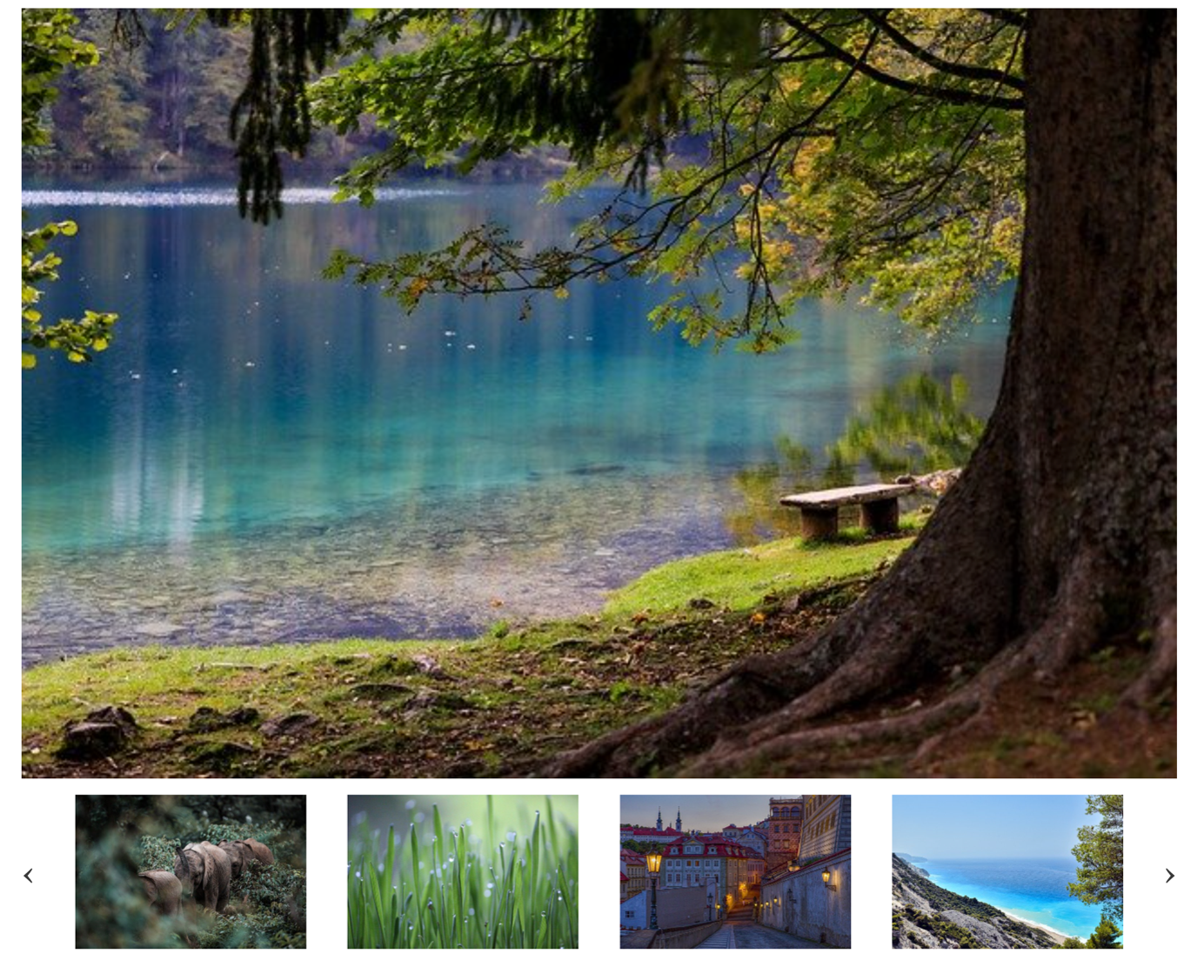
If you did not manage to complete, don't worry. This is all about gaining practice and experience. This lesson contains a solution to the project. There is no right or wrong way to do this, this is just one way to create the project.
Solution
All of the code for this project was added to the carousel.js
file:
// Create an arrar of image file paths
let images = [
"carousel/images/beach.jpg",
"carousel/images/elephants.jpg",
"carousel/images/grass.jpg",
"carousel/images/lake.jpg",
"carousel/images/town.jpg",
];
// Store a reference to the carousel element
const wrapper = document.querySelector("#carousel");
// The setImage function will loop over the images array, and
// set the CSS classes to structure the carousel.
function setImages() {
wrapper.innerHTML = "";
const smallImageWrapper = document.createElement("div");
smallImageWrapper.id = "smallImageWrapper";
images.forEach(function (imageSrc) {
let element = document.createElement("img");
element.src = imageSrc;
if (!wrapper.hasChildNodes()) {
element.classList.add("large", "carousel");
} else {
element.classList.add("small", "carousel");
element.addEventListener("click", swapImages);
smallImageWrapper.appendChild(element);
element = smallImageWrapper;
}
wrapper.appendChild(element);
});
setArrows();
}
// The reOrder function checks if the left or right arrow was clicked. We loop over the
// existing images, re-arrange the index positions, and create a modified array.
function reOrder(e) {
let newArray = [];
const direction = e.target.id;
images.forEach(function (image, index) {
if (direction === "left") {
if (index === 0) {
newArray[images.length] = image;
newArray.shift();
} else {
newArray[index - 1] = image;
}
} else {
newArray[index + 1] = image;
if (index + 1 === images.length) {
newArray[0] = image;
newArray.pop();
}
}
});
images = newArray;
setImages();
}
// The setArrows function creates the arrows to cycle through the images
function setArrows() {
const leftArrow = document.createElement("span");
leftArrow.innerHTML = "‹";
leftArrow.id = "left";
leftArrow.addEventListener("click", reOrder);
const rightArrow = document.createElement("span");
rightArrow.innerHTML = "›";
rightArrow.id = "right";
rightArrow.addEventListener("click", reOrder);
const smallImageWrapper = document.querySelector("#smallImageWrapper");
smallImageWrapper.appendChild(rightArrow);
smallImageWrapper.prepend(leftArrow);
}
// swapImages allows a small image to be clicked on and
// replaces the large image by mving it to beginning of the array
function swapImages(e) {
if (e.target.classList.contains("large")) return;
const imgSrc = e.target.getAttribute("src");
const selectedImageIndex = images.indexOf(imgSrc);
const firstImage = images[0];
images[0] = images[selectedImageIndex];
images[selectedImageIndex] = firstImage;
setImages();
}
setImages();
Congratulations!
We hope you have enjoyed this challenge and gained some valuable practice. You have now completed the JavaScript course!